Learning Unity — Taking Audio To The Next Level In Unity

In the last article, we dipped our toes into the Unity audio system and learned how to get audio clips to play in our Unity games. In this article, we’ll be taking things one step further with audio and learn how to make specific audio clips play when we want them to through scripting. Let’s get started!
First we’ll need an open Unity project and a couple of audio assets imported. Any audio is fine, but for this example I would recommend clips that are short and distinct so that we can hear the difference.
Next we will require a game object to originate the audio from. This could be any kind of game object, empty or not. Once we have created the object, we will then need to add the Audio Source component to the object.

Within the Audio Source component, make sure to unselect the Play On Awake and Loop options if they are presently selected. Next, find the AudioClip option and fill that field with one of the imported audio assets.
With the Audio Source component now configured, we are ready to begin working on our script. Create a script, attach it to the game object, then open it in a code editor.
The code to play the specified audio clip is really quite simple. It only has two steps. First, access the Audio Source component; and second, run the Play method.
GetComponent<AudioSource>().Play();
That line of code, placed appropriately in your script, will play the audio clip specified in the Audio Source. Like with other scripting examples my articles have covered in the past, we could have the audio play under any number of different conditions. For example, it could be triggered by a collision or a certain amount of time passing. Check out some of my previous articles to see how you could have the audio triggered through user input.
Now the game will play the audio clip at a point specified in our script. That’s good, but you might have noticed an issue with this approach. What issue is that? Well, our Audio Source only takes one audio clip as a parameter. What if we had multiple sounds for this game object to play?
This is fairly standard in modern games. An enemy character might have both a battle cry and a death cry, or even multiple versions of both. A player character in an RPG could have dozens of different clips for taunts, quips, battle cries, and more. So how do we handle this?
Let’s return to the script we just created. Instead of relying on the Audio Source to store the reference to our audio clip, we will need to create a script level variable for every audio clip the game object needs access to. Make use of the SerializeField attribute to more easily reference the appropriate clips.
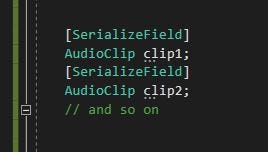
Once we have the audio clip references, we can switch these in the Audio Source component by updating the clip property.
GetComponent<AudioSource>().clip = clip1;
This line updates the Audio Source’s audio clip, so when the Play method is invoked the updated clip is played. That’s all there is to it!
That wraps up our work with Unity audio for the time being. In the next article, we’re going to learn how to build our games so they don’t have to be run through the Unity Editor. Until then, good luck and happy coding!